Stencil and Blend Tutorial
WebGL Techniques
Overview
This tutorial explains how to apply WebGL blending with WebGL stencils. The example project, Stencil Blend Visual Tool, displays a background graphic, a three dimensional cube, and a stencil. A portion of the background graphic displays where the stencil masks part of the cube. The stencil applies both transparent and non transparent areas of a texture from a GIF image file.
Each side of the cube is texture mapped with a different color. A large quad renders the background graphic behind the cube. A small stationary stencil with transparent bars masks the cube. As the cube passes over the stencil area, blend options apply to the stencil. See a portion of the background quad through the cube, where the stencil masks the cube. Try different settings from the blend menus to see how blend values modify rendering of the stencil.
See the Stencil with Transparent Bars. See the Cube Mapped Texture. See the Background Area Quad which is applied as a texture map to a square flat plane for the background.
See All Graphics
The following two images provide a good overview of the graphics applied to the Stencil Blend Visual Tool. Textures include a background desert photograph, bars with transparency and solid colored rectangles for the cube. Screen shots include views from the Stencil Blend Visual Tool, labeled with menu selections.
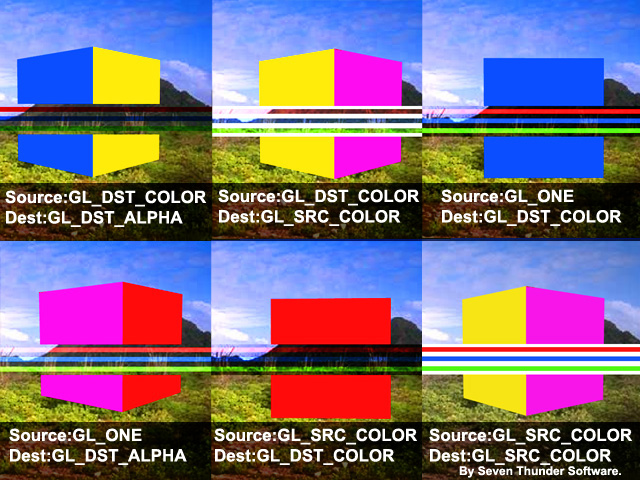
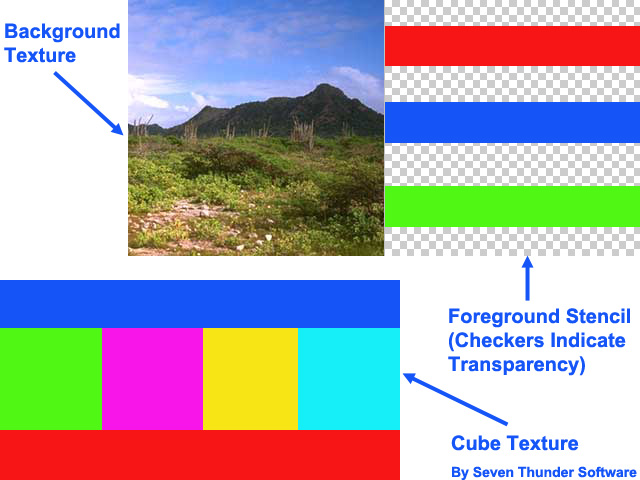
WebGL Blend and Stencil JavaScript
The code to draw the background graphic, stencil, and cube with blending, includes six steps. First render the background. Second clear the stencil buffer. Third prepare to draw the stencil. Fourth enable blending. Fifth draw the stencil. Sixth disable blending and draw the interactive cube. The following paragraphs describe each step.
First render the background.
Assign the background graphic's
texture unit to the shader's Sampler2D
.
Variable uSampler
in the following
listing is the Sampler2D
in a simple
fragment shader.
Variable eLast.idx
is an integer
which represents the
texture unit for the background area quad.
gl.uniform1i ( uSampler, eLast.idx );
Render the background graphic.
Method renderQuad()
in the following listing
simply renders a square, mapped edge to edge with
the background area quad.
glUtils.renderQuad( controller, eLast );
Second clear the stencil buffer with the following
two WebGL methods.
gl
is a reference of WebGLRenderingContext
.
gl.clearStencil( 0 ); gl.clear( gl.STENCIL_BUFFER_BIT );
Third prepare to draw the stencil.
Call the WebGL stencilFunc()
.
gl.stencilFunc( gl.ALWAYS, 1, 1 );
Call WebGL method stencilOp()
to replace the current stencil
with the result returned from stencilFunc()
.
gl.stencilOp( gl.REPLACE, gl.REPLACE, gl.REPLACE );
Fourth enable blending. Call gl.enable(gl.BLEND)
.
Call the WebGL blendFunc()
, based
on source and destination values assigned through
blend menus.
In the following listing
properties glSource
and glDest
represent WebGL constant Source Options
and Destination Options.
JavaScript also enables the stencil test function
with the WebGL method enable()
.
gl.enable( gl.BLEND ); gl.blendFunc( glDemo.glSource, glDemo.glDest ); gl.enable( gl.STENCIL_TEST );
Fifth draw the stencil.
Activate the stencil's texture unit.
Upload the stencil's transformation
matrix, and draw the stencil.
Variable eSmallSquare
in the following
listing is an entity which represents the
square that displays the stencil bars.
Property eSmallSquare.idx
is
the texture unit for the stencil texture.
Property
eSmallSquare.matrix
is the
matrix for the stencil.
gl.uniform1i ( uSampler, eSmallSquare.idx ); gl.uniformMatrix4fv ( controller.uMatrixTransform, false, new Float32Array ( eSmallSquare.matrix ) ); gl.drawElements ( gl.TRIANGLES, eSmallSquare.nCount, gl.UNSIGNED_SHORT, eSmallSquare.nOffset );
Sixth disable writing to the stencil. Disable blending then draw the interactive cube.
gl.stencilFunc( gl.EQUAL, 1, 1 ); gl.stencilOp( gl.KEEP, gl.KEEP, gl.KEEP ); gl.disable( gl.STENCIL_TEST ); // Display the // actual colors. // Stencil prevents // drawing to // transparent areas. gl.disable( gl.BLEND ); gl.colorMask( true, true, true, true ); //Activate the //cube texture. gl.uniform1i ( uSampler, eCube.idx ); glUtils.renderInteractiveXY( controller, eCube );
Summary WebGL Blend and Stencil JavaScript
The code to draw the background graphic, stencil, and cube with blending, includes six steps. First render the background. Second clear the stencil buffer. Third prepare to draw the stencil. Fourth enable blending. Fifth draw the stencil. Sixth disable blending and draw the interactive cube.
Blending Options
The WebGL blendFunc()
includes a number of constants to apply as
source and destination blending parameters.
In the following
listing assign options to the menuSourceSelection
parameter from the list of source blending selections.
Assign options to the menuDestSelection
parameter from the list of destination blending selections.
Source Options
The following list includes source selections for WebGL blending. Each option is a WebGL constant.
GL_ONE GL_SRC_COLOR GL_DST_COLOR GL_DST_ALPHA GL_SRC_ALPHA GL_ONE_MINUS_SRC_ALPHA GL_ONE_MINUS_SRC_COLOR GL_ONE_MINUS_DST_COLOR GL_ONE_MINUS_DST_ALPHA GL_CONSTANT_COLOR GL_ONE_MINUS_CONSTANT_COLOR GL_CONSTANT_ALPHA GL_ONE_MINUS_CONSTANT_ALPHA GL_SRC_ALPHA_SATURATE GL_ZERO
Destination Options
The following list includes destination selections for WebGL blending. Each option is a WebGL constant.
GL_ONE GL_SRC_COLOR GL_DST_COLOR GL_DST_ALPHA GL_SRC_ALPHA GL_ONE_MINUS_SRC_ALPHA GL_ONE_MINUS_SRC_COLOR GL_ONE_MINUS_DST_COLOR GL_ONE_MINUS_DST_ALPHA GL_CONSTANT_COLOR GL_ONE_MINUS_CONSTANT_COLOR GL_CONSTANT_ALPHA GL_ONE_MINUS_CONSTANT_ALPHA GL_ZERO
Summary
This tutorial explained how to apply WebGL blending with WebGL stencils. The example project, Stencil Blend Visual Tool, displays a background graphic, a three dimensional cube, and a stencil. A portion of the background graphic displays where the stencil masks part of the cube. The stencil applies both transparent and non transparent areas of a texture from a GIF image file.
Each side of the cube is texture mapped with a different color. A large quad renders the background graphic behind the cube. A small stationary stencil with transparent bars masks the cube. As the cube passes over the stencil area, blend options apply to the stencil. See a portion of the background quad through the cube, where the stencil masks the cube. Try different settings from the blend menus to see how blend values modify rendering of the stencil.
Graphics
Background Area Quad
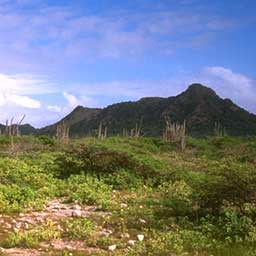
Stencil Transparent Bars Display the White Page Background

Cube Mapped Texture
