HTML Elements
Page 3
Scaleable Image Map
Introduction
Learn to create an HTML image map that scales with the user's device. Coordinates are smaller for phones and larger for desktop computers. See the next page for a simpler image map example.
Display an image in HTML and associate the image with a map name.
The image includes a usemap
attribute and value.
<img...usemap="#brochure">
.
The map's name matches the image's usemap
value.
<map name="brochure">
This example applies an image that's small enough to look good on a mobile phone. However you can scale elements up for larger screens, with JavaScript and CSS. Control the scale amount with preset constant arrays of coordinates. The arrays may take a little more memory but they save processsing time.
Image Map
Outside of a Brochure
Tap Items on the Image
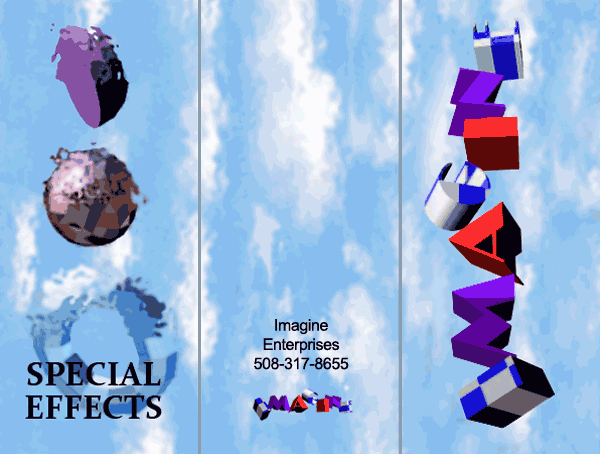
Image Markup
The following markup displays the image at the smaller dimension; 300 pixels wide by 227 high. However see the CSS section where dimensions for smaller devices and desktop computers are specified.
<img id="imMap" width="300" height="227" src="brochure-outside.png" alt="outside of brochure" usemap="#brochure" >
Map Markup
The usemap
attribute declared with
the image, above, matches the name
attribute in the map
below, as brochure
.
The following markup shows the smaller coordinates when a mobile phone or small tablet
displays the image.
Please notice that each area
includes
a specific onclick
event and id
attribute-value pair.
The events, in this example simply tell the user what they tapped.
However onclick
events can accomplish a wide variety of tasks
when the user taps an image area.
See the
Respond to Image Area Clicks
for details.
Later you'll see, JavaScript accesses each area
tag by the id
attribute
in order to modify coordinates.
<map name="brochure"> <area id="c" shape="circle" coords="45,85,22" alt="Sphere" onclick="tappedSphere()" href="#eDebug" > <area id="logoLg" shape="rect" coords="200,0,296,225" alt="Logo" onclick="tappedLogo()" href="#eDebug" > <area id="logoSm" shape="rect" coords="112,194,183,224" alt="Logo" onclick="tappedLogo()" href="#eDebug" > <area id="address" shape="rect" coords="120,155,172,185" alt="Address" onclick="tappedAddress()" href="#eDebug" > <area id="nothing" shape="rect" coords="96,0,200,153" alt="Clouds" onclick="tappedNothing()" href="#eDebug" > <area id="effect" shape="rect" coords="0,175,84,214" alt="Torus Effect" onclick="tappedEffect()" href="#eDebug" > <area id="hemi" shape="poly" coords="19,12,40,67,49,71,59,9" alt="Hemisphere" onclick="tappedHemisphere()" href="#eDebug" > </map>
JavaScript
JavaScript scales coordinates up or down based on the content area's width. When the Web page loads, the user rotates their device or resizes the browser window, then coordinates for each area are scaled.
Variables
Reusable variables include the eDebug
element for displaying output,
and the e
element to obtain the page's main content width, during resize events.
Also, of course, note the rather large const
arrays
with coordinates.
Arrays allow simple for loops to resize the image coordinates. Just
read the array and assign coordinates to each element.
var eDebug = null; // Element with all content: var e = null; // Array for all area elements: var aElements = null; // Start the image at full size: var bScaledDown = false; // Coordinates for each element // in order; // c,logoLg,logoSm,address,nothing,effect,hemi: const aCoordsSmall = ["45,85,22", "200,0,296,225","112,194,183,224", "120,155,172,185","96,0,200,153", "0,175,84,214", "19,12,40,67,49,71,59,9"]; const aCoordsLarge = ["89,194,95","398,0,598,451","240,390,356,428", "238,312,345,375","189,0,410,308","22,281,178,418", "29,28,82,139,144,99,102,8"];
Load The App
First assign loadExample
and resizeCoords()
within the body tag.
<body onload="loadExample()" onresize="resizeCoords()" >
When the page loads, then access and save elements by id
attributes.
Each area element is saved within the
array named, aElements
.
Array aElements
stores each area element
in the exact same order, as the two coordinate arrays, above,
named aCoordsSmall
and aCoordsLarge
.
/*** * When the page loads * save the eDebug element * for output. * Save all areas to scale up or down. */ function loadExample(){ eDebug = document.getElementById('eDebug'); // Save area elements: //Content element. e = document.getElementById('e'); //Circle var c = document.getElementById("c"); //Large logo var logoLg = document.getElementById("logoLg"); //Small logo var logoSm = document.getElementById("logoSm"); //Address text var address = document.getElementById("address"); //Clouds var nothing = document.getElementById("nothing"); //Torus effect: var effect = document.getElementById("effect"); //Hemisphere: var hemi = document.getElementById("hemi"); // Array for resizing: aElements = [c,logoLg,logoSm,address,nothing,effect,hemi]; // Resize image map if needed: if(e.clientWidth > 600){ scaleUp(); } } /*** * Scale up or scale down * based on the client's width. * consider a constant for the * scale width such as * const S_WIDTH_MAX = 600; */ function resizeCoords(){ if(e.clientWidth < 600) scaleDown(); else scaleUp(); }
Scaling the Image Map
When the page loads save the set of area elements in
array, aElements
, just once.
Also predefine two constant arrays of coordinates.
Declare an array for the smaller image size. The array's named aCoordsSmall
.
Declare an array for the larger image size. The array's named aCoordsLarge
.
The coordinates themselves were obtained from Photoshop's info feature.
The boolean bScaledDown
variable,
prevents multiple scaling at the same size.
/*** * Scale every area down. */ function scaleDown(){ if (bScaledDown == true) return; for (j = 0; j < aElements.length; j++){ var elem = aElements[j]; var coords = aCoordsSmall[j]; elem.coords = coords; } bScaledDown = true; } /*** * Scale every area up. */ function scaleUp(){ if (bScaledDown == false) return; for (j = 0; j < aElements.length; j++){ var elem = aElements[j]; var coords = aCoordsLarge[j]; elem.coords = coords; } bScaledDown = false; }
Respond to Image Area Clicks
When the user taps an area of the image, then
specific JavaScript functions activate.
For example click the sphere to activate
function tappedSphere()
.
Function tappedSphere()
activates when the circular
sphere area of the brochure's tapped, as follows.
<area id="c" shape="circle" coords="45,85,22" alt="Sphere" onclick="tappedSphere()" >
Function tappedSphere()
simply displays
which part of the image the user clicked.
However many more interesting and useful Web pages can be created with image maps.
The other areas, in this example, respond with similar functions.
/*** * If the user selects the * sphere, let them know. */ function tappedSphere(){ if(eDebug != null){ eDebug.innerHTML = "You selected the sphere."; } }
CSS
You've seen inline styles within markup, in previous examples. The following short listing applies a media query to scale the image down, in portrait mode. The default leaves the image scaled up. Notice width and height receive precise pixel width and height values. These values are necessary to respond to exact coordinate settings.
<style> #imMap{ width:600px; height:454px; display:block; margin-left:auto; margin-right:auto; text-align:center; } @media (orientation: portrait) { #imMap{ width:300px; height:227px; } } </style>
Summary
You learned to create an HTML image map that scales with the user's device. Coordinates are smaller for phones and larger for desktop computers. See the next page for a simpler image map example.
Display an image in HTML and associate the image with a map name.
The image includes a usemap
attribute and value.
<img...usemap="#brochure">
.
The map's name matches the image's usemap
value.
<map name="brochure">
This example applied an image that's small enough to look good on a mobile phone. However you can scale elements up for larger screens, with JavaScript and CSS. Control the scale amount with preset constant arrays of coordinates. The arrays may take a little more memory but they save processsing time.
Unusual HTML5 Skills
See the markup, CSS and results of various HTML5 elements, with styles and some unusual or seldom used techniques.