JavaScript: Change Content, Loops, Statements, Identifiers
Page 5
Change Content, Loops, Statements, Identifiers
Introduction
Learn to change Web page content, run various loops, display, close and style new windows. Read an overview of JavaScript statements, fixed values, naming and rules conventions for identifiers.
Change Content
Learn to change content on a Web page including images, HTML elements, input elements, the entire Web page, the Web developer console, an alert and window popups.
Loops
Learn about loops including
for, for-in, for-of, while
and do-while
iteration.
JavaScript Source Code
See the source code for this page. Read JavaScript file loops.js.
Change Content
Tap to Change Image
The img.src
property
alternates between two different
images, when you tap the image below.
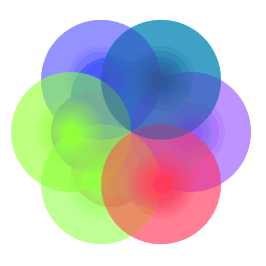
Source Code to Change Image
The following JavaScript alternates
the image source on display, in HTML
element id, im
.
var bImgChanged = false; function changeImg(){ let im = null; if(im == null){ im = getElement('im'); } if(bImgChanged){ im.src="../../thumbnails/ss-rga.png"; bImgChanged = false; } else{ im.src="../../thumbnails/ss-meter-range.png"; bImgChanged = true; } }
HTML Element Content
Change input element text with the
value
property.
Change the text on most other types of HTML elements, with the
innerHTML
property.
function changeHTML(sId){ let e = getElement(sId); e.innerHTML = "Changed!"; }
function changInput(sId){ let e = getElement(sId); e.value ="Changed!"; }
Change Document Content
Write directly to this document
and overwrite the entire page.
Tap the
aqua colored box below,
to display Changed Document!
, in place
of this page's content.
Re-load this page to continue reading.
Write to the Console
Open your Web browser's debugger. For example with FireFox select the upper right menu:
- Select
More Tools>Web Developer Tools
. - Select the
Console
tab. - Tap the
logs
tab. - Tap the violet button below to see
Changed log!
in your console tab.
function changeDoc(){ document.write("Changed!"); }Click to Change Document!
function changeOutput(){ console.log("Changed Log!"); }Click to Change Console Log!
Window Methods
Learn to open, close and style windows.
Window Alert, Open, Close
Tap the orange box to display an alert window that renders above your Web page. Tap the violet box to open or close a 200 x 100 pixel window.
Tap a Box
alert('Showing Alert!');
var win = null; function winOpen(sBG) { if (win == null || win.closed){ win = window.open( "", "", "width=200,height=100" ); win.document.write("New Window!"); // Style this window: winStyleBG(sBG); } else{ win.close(); win = null; } }
Style a Window
Function winNewBG()
calls function winStyleBG()
in the following two examples.
If the small window's open then
winNewBG()
brings the current window to the
front and styles it.
Otherwise winNewBG()
creates a new window and styles it.
Please see functions
winNewBG()
and
winStyleBG()
in JavaScript file
loops.js.
Function winNewBG()
opens a new small window.
However if a window's already open then
winNewBG()
calls
winStyleBG()
to modify the current
window's style.
Function winStyleBG()
displayed
in the two boxes below,
applies JavaScript to modify
CSS values for properties
color, textAlign, fontWeight
and backgroundColor
, on
the open window.
Tap Boxes for Styled Windows
function winStyleBG(bg){ win.document.body.style.color = "white"; win.document.body.style.textAlign = "center"; win.document.body.style.fontWeight = "bold"; win.document.body.style.backgroundColor = bg; }
function winStyleBG(bg){ win.document.body.style.color = "white"; win.document.body.style.textAlign = "center"; win.document.body.style.fontWeight = "bold"; win.document.body.style.backgroundColor = bg; }
Tap Colored Boxes
Each colored box below, changes the small popup window's background color.
Each box calls function winStyleBG()
.
The arguments for each function range from
violet, yellow, orange, red
to purple
.
JavaScript Statements
JavaScript statements include values, operators, expressions, keywords and comments.
The previous Source Code to Change Image
section included an example of simple code.
Each statement ends in a semi-colon - ;
.
Statements should always end in a semi-colon.
The following line demonstrates a simple statement.
String This is a statement.
on the right,
is assigned to variable, s
, on the left.
var s = "This is a statement";
Expressions
An expression is a subset of a statement. A statement performs an action. Expressions contain values, variables and operators. Expressions evaluate to a specific value. An expression is a statement however not all statements are expressions.
The following example is an expression.
Variable x
evaluates to 110
.
Operator +
adds 10
to 100
.
var x = 10 + 100;
Fixed Values
Fixed values are also called literals. A literal cannot be changed. Fixed values include string, numeric, boolean, array, regular expression and even object literals. The following string is a literal.
I am a Web Developer.
The following digit is a numeric literal.
10
Usually a literal's assigned to a variable or
constant.
Constant N_TEN
is a constant.
Value 10
is a digital literal.
const N_TEN = 10;
Literal I write code.
is
assigned to variable s
, below.
s = "I write code.";
Tap a Box for Numbers & Strings
The dark green box applies numeric literal 10
to an expression.
The light green box displays string entries from
an array literal named a
, as follows.
let a = ["Cats","Dogs","Parrots"];
function changeNumber(sId){ let e = getElement(sId); e.innerHTML = Math.round( Math.random() * 10 ); }Tap Repeatedly -> New Numbers!
var k = 0; function changeString(sId){ let a = ["Cats","Dogs","Parrots"]; let l = a.length; let e = getElement(sId); e.innerHTML = a[k]; k++; if(k == l){ k = 0; } }Tap -> Displays One Word at a Time.
Indentifier Rules & Conventions
Create identifiers to name variables and functions.
Rules
The first character of a identifier must contain a letter, underscore or dollar sign. Subsequent letters can contain letters, underscores, dollar signs or digits.
With JavaScript you can declare variables on one line, separated by a comma, as follows.
var cat = "Bombay", catType = "Domestic", neuterCost = 100;
Restrictions
You can't use a number as the first character.
Plus JavaScript identifiers are case sensitive.
Therefore cow
and Cow
represent different elements.
You saw examples of identifiers in the section titled,
Source Code to Change Image.
For example variables im
and bImgChanged
were identifiers.
The example code was prepared to be concise.
However often you'll want very legible, self describing
code. That allows other developers to understand your
code easier.
Camel Case Convention
Camel case naming's often applied to function names
and variable identifiers.
Camel case separates the first character
of words with upper case letters,
yet camel case names begin with lower case letters.
For example brownCat
and windowPopup
,
begin words brown
and window
in
lower case. The second words, Cat
and Popup
are easy to differentiate
with upper case letters.
Verb-Noun Function Convention
Functions are often named with two words. The first word is a verb, describing the function's action. The second word is a noun, describing which elements the function acts on.
Loops - For, For In, For of, While, Do While
JavaScript loops allow developers to execute one code block
multiple times. Loops include
for, for in, for of, while
and do while
.
Tap boxes below and see loops.js for each loop type's implementation.
For Loop
The for loop increments or decrements a variable until that variable reaches a coded termination value.
The variable, j
, in the following
example starts at one, increments by one for
each loop and exits at three.
for(let j = 1; j < 4; j++){...}
For In Loop - Associative Array
Associative arrays include attribute:value
pairs.
Each attribute's associated with a value.
The following associative array includes
attributes
generic, type
and coat
.
The following associative array includes
values
Wild
, Jaguar
and Spotted
.
const cat = { generic:"Wild", type:"Jaguar", coat:"Spotted" };
The for in
loop iterates over each value
in an associative array. The for in
loop
ignores each attribute in an associative array.
The for in
loop returns only values.
For Loop
The for
loop iterates over
a set number of values.
The example for
loop, in the violet box,
loops from digits zero to three.
The for - in
loop, in the yellow box
iterates over a set of associative array entries,
displaying just the entry values.
Tap a Box: For Loop, For in Loop
For Loop
for(let i = 0; i < 4; i++){ dFor.innerHTML += "<br>i: " + i; }
For In Loop
// Associative array: const cat = { generic:"Wild", type:"Jaguar", coat:"Spotted" }; // Access by index: for (let c in cat) { dForIn.innerHTML += cat[c] +"<br>"; }
For Of Loop
The for of loop iterates over a simple array, retrieving each element in the array. The loop stops when all elements in an array have run through the loop.
While Loop
The while loop runs during the
time a specified condition returns true.
Therefore the following while loop,
code snippet,
will run forever because the
condition always equals true
.
Be sure to include
a terminating value.
The loop must stop at some specified criteria.
while (true){....}
For example,
while (i < 4)
will run until i
is greater than
or equal to four. In other words, if i
starts
at four or above, the loop never runs.
If i
increments during the while loop,
when i
equals four or exceeds four, the loop
stops running.
At some point
i
must be greater than three to
exit the loop.
Tap a Box: For Of, While Loops
// Simple array: const cats = ["Siamese","Bombay","Lynx"]; for (let c of cats){ // Access by element dForOf.innerHTML += c +"<br>"; }
let i = 0; while (i < 4){ dWhile.innerHTML += "i:"+i+"<br>"; // Increment i in this loop: i++; }
Do While Loop
Learn to apply JavaScript do-while, from ECMAScript 3.
The do-while loop will always run atleast once. The loop runs, then checks an exit condition at the end of the loop.
Tap a Box: Two Do While Loops
The blue box will run once,
despite the fact that i
starts at 0
.
The blue box displays
0
, then exits because the test
for (i < 0)
processes
at the end of the loop.
The violet box runs while i
equals
0,1,2,3
and 4
,
exiting when i
equals 5
.
let i = 0; do{ doWhile.innerHTML += "i:"+i+"<br>"; i++; } while (i < 0)
Do While Loop
let i = 0; do{ doWhile.innerHTML += "i:"+i+"<br>"; i++; } while (i < 5)
Summary
You learned how to change Web page content, run various loops, display, close and style new windows. You read an overview of JavaScript statements, fixed values, naming and rules conventions for identifiers.
Change Content
You learned to change content on a Web page including images, HTML elements, input elements, the entire Web page, the Web developer console an alert and window popups.
Loops
You learned about loops including
for, for-in, for-of, while
and do-while
iteration.
JavaScript Source Code
See the source code for this page in JavaScript file loops.js.
Learn JavaScript
JavaScript's the foundation of Web developer and Website design skills. This free and unique JavaScript tutorial includes some new or seldom used, but useful features.